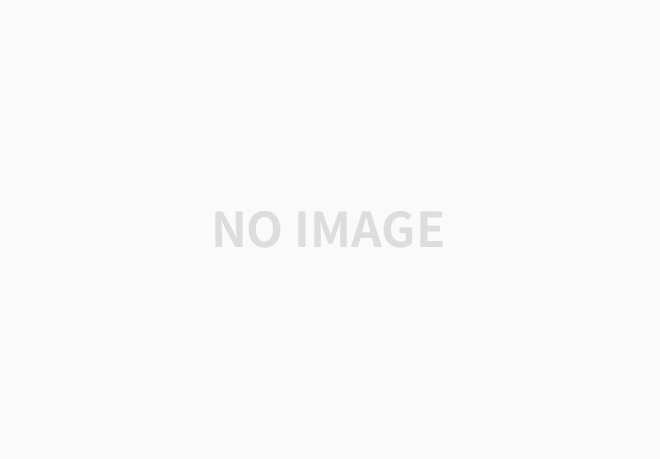
/*
* main3_4pins.c
*
* Created: 2020-11-09 오후 1:57:06
* Author: User
*/
#define F_CPU 16000000UL
#include <avr/io.h>
#include <util/delay.h>
#define LCD_DATA_DIR DDRD
#define LCD_CTRL_DIR DDRB
#define LCD_DATA_PORT PORTD
#define LCD_CTRL_PORT PORTB
#define RS PORTB0
#define EN PORTB1
void lcdComm(unsigned char cmd){
//상위 4비트 처리
LCD_DATA_PORT = (LCD_DATA_PORT & 0x0F) | (cmd & 0xF0);
LCD_CTRL_PORT &= ~(1 << RS);
LCD_CTRL_PORT |= (1 << EN);
_delay_us(1);
LCD_CTRL_PORT &= ~(1 << EN);
//하위 4비트 처리
LCD_DATA_PORT = (LCD_DATA_PORT & 0x0F) | (cmd << 4);
LCD_CTRL_PORT |= (1 << EN);
_delay_us(1);
LCD_CTRL_PORT &= ~(1 << EN);
//_delay_ms(2);
_delay_us(1530);
}
void lcdChar(unsigned char data){
//상위 4비트 처리
LCD_DATA_PORT = (LCD_DATA_PORT & 0x0F) | (data & 0xF0);
LCD_CTRL_PORT |= (1<<RS);
LCD_CTRL_PORT |= (1<<EN);
_delay_us(1);
LCD_CTRL_PORT &= ~(1<<EN);
//하위 4비트 처리
LCD_DATA_PORT = (LCD_DATA_PORT & 0x0F) | (data << 4);
LCD_CTRL_PORT |= (1<<EN);
_delay_us(1);
LCD_CTRL_PORT &= ~(1<<EN);
_delay_ms(2);
}
void lcdInit(){
_delay_ms(30); //Wait for more than 30ms after VDD rises to 4.5V
lcdComm(0x02); // init 4bit mode로 세팅 (전원 인가 후 기본 8비트 모드로 동작)
lcdComm(0x28); // function set
lcdComm(0x0F); // display on/off
lcdComm(0x01); // display clear
lcdComm(0x06); // entry mode set
}
void lcdStr(char *str){
for(int i=0; str[i]!=0; i++){
lcdChar(str[i]);
}
}
void lcdStrPos(int row, int pos, char *str){
#if 0
if (row == 0 && pos<16){
lcdComm((pos & 0x0F) |0x80);
}
else if (row == 1 && pos<16){
lcdComm((pos & 0x0F)| 0xC0);
}
#else
lcdComm(0x80 | (pos + row * 0x40));
#endif
lcdStr(str);
}
void lcdClear(){
lcdComm(0x01);
}
int main(){
LCD_CTRL_DIR = (1 << RS | 1 << EN);
LCD_DATA_DIR = 0xF0;
lcdInit();
lcdStrPos(0, 4, "delay1530"); //첫번째 줄 5번째 칸 부터 출력
lcdStrPos(1, 2, "2NE LINE"); // 두번째 줄 3번째 칸 부터 출력
while(1);
}
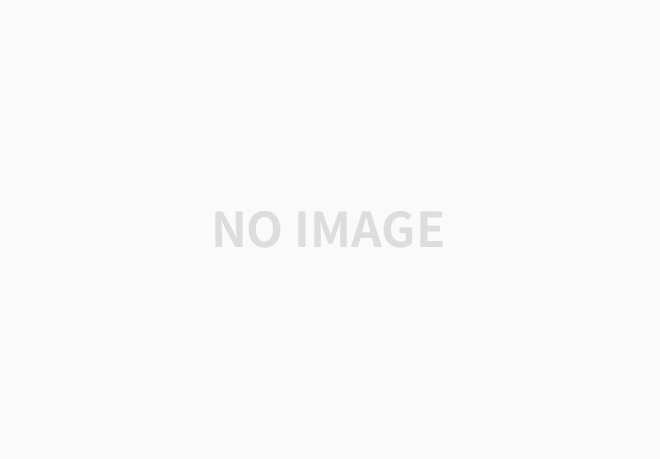
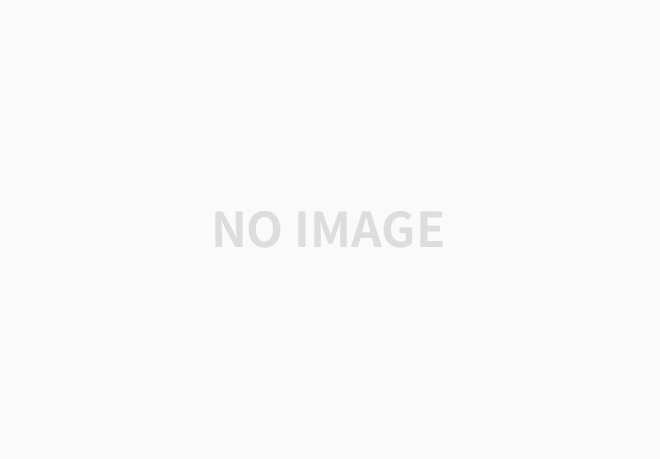
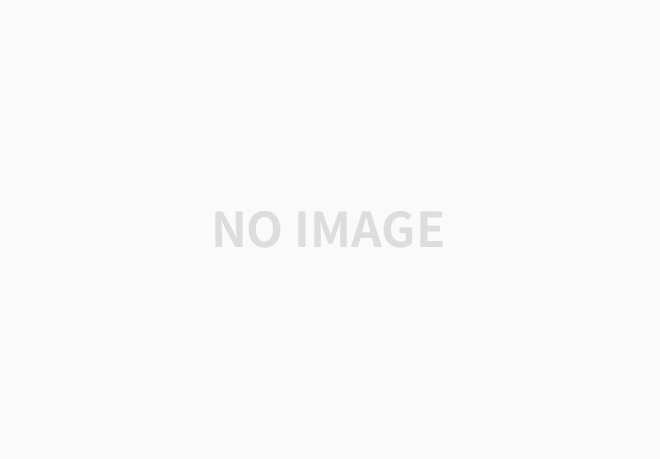
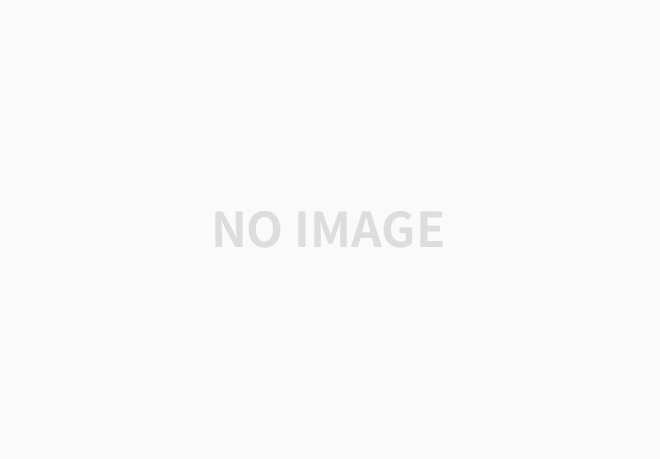
'임베디드 > Atmega328p' 카테고리의 다른 글
ATmega328p datasheet (0) | 2020.12.17 |
---|---|
16x2 8핀제어 데이터시트 그대로 적은 코드 (0) | 2020.11.09 |
16x2 LCD D0, D1 -> C 포트에 꼽은 코드 (0) | 2020.11.07 |
16x2 LCD (LC1621-SMLYH6) (0) | 2020.11.06 |
interrupt 2개 사용 LED 2개 제어 (0) | 2020.11.06 |